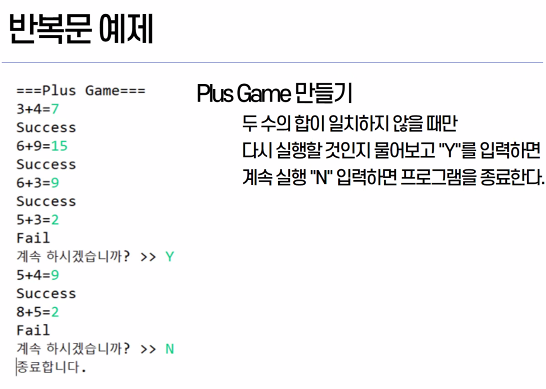
728x90
// 랜던 숫자 출력하는 기능 가져오기
Random rd = new Random();
// 랜덤 숫자 출력해보기
int num = rd.nextInt();
System.out.println(num);
// 랜덤 숫자의 범위를 설정(0-9사이의 랜덤한 숫자)
int num2 = rd.nextInt(10); // 0부터 적혀있는 숫자에서 -1까지
System.out.println(num2);
// 1-10까지의 랜덤한 숫자를 출력
int num3 = rd.nextInt(10)+1;
System.out.println(num3);
■While문 예제
예제1) Plus Game 만들기
정답)
package while문예제;
import java.util.Random;
import java.util.Scanner;
public class ex02while문예제 {
public static void main(String[] args) {
// Plus Game 만들기
// 입력하는 도구 가져오기
Scanner sc = new Scanner(System.in);
// 랜덤숫자 출력하는기능
Random rd = new Random();
// *import 단축키 : ctrl + shift + o
// 1. 출력문
System.out.println("===Plus Game===");
// 2. 문제 출력(문제는 랜덤한 숫자로 이루어진 식)
// 3. 사용자에게 정답 입력받기
// 4. Success/Fail
// : 연산에 대한 결과값과 사용자가 입력한 정답이
// 같으면 --> Success
// 다르면 --> Fail
while(true) {
int num1 = rd.nextInt(50)+1;
int num2 = rd.nextInt(50)+1;
System.out.print(num1+"+"+num2+"=");
int res = sc.nextInt();
if(num1+num2 == res) {
System.out.println("Success");
}else {
System.out.println("Fail");
System.out.print("계속 하시겠습니까? >> ");
String ans = sc.next();
if(ans.equals("N")) {
// 숫자 비교 : ==
// 문자 비교 : .equals()
System.out.println("종료합니다.");
break;
}
}
}
}
}
예제2)
정답)
package while문예제;
import java.util.Random;
import java.util.Scanner;
public class ex03while문예제 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Random rd = new Random();
int ran = rd.nextInt(100)+1;
System.out.println("======1부터 100사이 숫자 맞추기 게임!======");
while(true) {
System.out.print("1과 100사이의 정수를 입력하세요 >> ");
int num = sc.nextInt();
if(num == ran) {
break;
}
}System.out.println("프로그램 종료");
}
}
추가문제)
package while문예제;
import java.util.Random;
import java.util.Scanner;
public class ex03while문예제 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Random rd = new Random();
int ran = rd.nextInt(100)+1;
System.out.println("======1부터 100사이 숫자 맞추기 게임!======");
while(true) {
System.out.print("1과 100사이의 정수를 입력하세요 >> ");
int num = sc.nextInt();
if(num>ran) {
System.out.println("더 작은 수로 다시 시도 해보세요");
}else if(num<ran) {
System.out.println("더 큰 수로 다시 시도 해보세요");
}else if(num == ran) {
System.out.println(ran+" 정답입니다!!");
break;
}
}System.out.println("프로그램 종료");
}
}
■이중 for문
-순서
예제1)
package 이중for문;
public class ex02이중for문 {
public static void main(String[] args) {
// *****
// *****
// *****
// *****
// *****
// 출력해보자
for(int i=1; i<=5; i++) {
for(int j=1; j<=5; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
예제2)
-결과 로직
package 이중for문;
public class ex03이중for문 {
public static void main(String[] args) {
for(int i = 2; i<=9; i++) {
System.out.print(i+"단 : ");
for(int j =1; j<=9; j++) {
System.out.print(i+"*"+j+"="+(i*j)+" ");
}System.out.println();
}
}
}
예제3)
package 이중for문;
public class ex04이중for문 {
public static void main(String[] args) {
for(int j=1; j<=5; j++) {
for(int i= 1;i<=j;i++) {
System.out.print("*");
}System.out.println();
}
}
}
예제3 변형1)
package 이중for문;
public class ex05이중for문 {
public static void main(String[] args) {
// *****
// ****
// ***
// **
// *
for(int j=5; j>=1; j--) {
for(int i=1; i<=j; i++) {
System.out.print("*");
}
System.out.println();
}
}
}
예제3 변형2)
package 이중for문;
public class ex06이중for문 {
public static void main(String[] args) {
// *
// **
// ***
// ****
//*****
for(int i= 1; i<=5; i++) {
for(int j=4; j>=i; j--) {
System.out.print(" ");
}
for(int k=1; k<=i; k++) {
System.out.print("*");
}
System.out.println();
}
}
}
* 몇번틀렸다. 띄어쓰기부분 안에 " * "부분 반복문을 넣어버려서 오류가 났다
별개로 for문은 두어야 결과화면처럼 나왔다.
범위도 j>=i, k>=i처럼 두어야하지만 어떻게 해야되는지 몰랐다.
i 로 동일하게 해야 한다.
_ | _ | _ | _ | * |
_ | _ | _ | * | * |
_ | _ | * | * | * |
_ | * | * | * | * |
* | * | * | * | * |
ps. 반복적인 구문을 찾아 만들고 후에 빌드업해서 만들자
728x90
'Study > JAVA' 카테고리의 다른 글
JAVA 기초 7일차 <배열> (1) | 2022.09.21 |
---|---|
JAVA 기초 6일차 <배열> (0) | 2022.09.19 |
JAVA 기초 4일차 <반복문(while문)> (1) | 2022.09.16 |
JAVA기초 3일차 <반복문(for문)> (0) | 2022.09.15 |
JAVA기초 2일차 <조건문> (0) | 2022.09.14 |